Guide: Forms
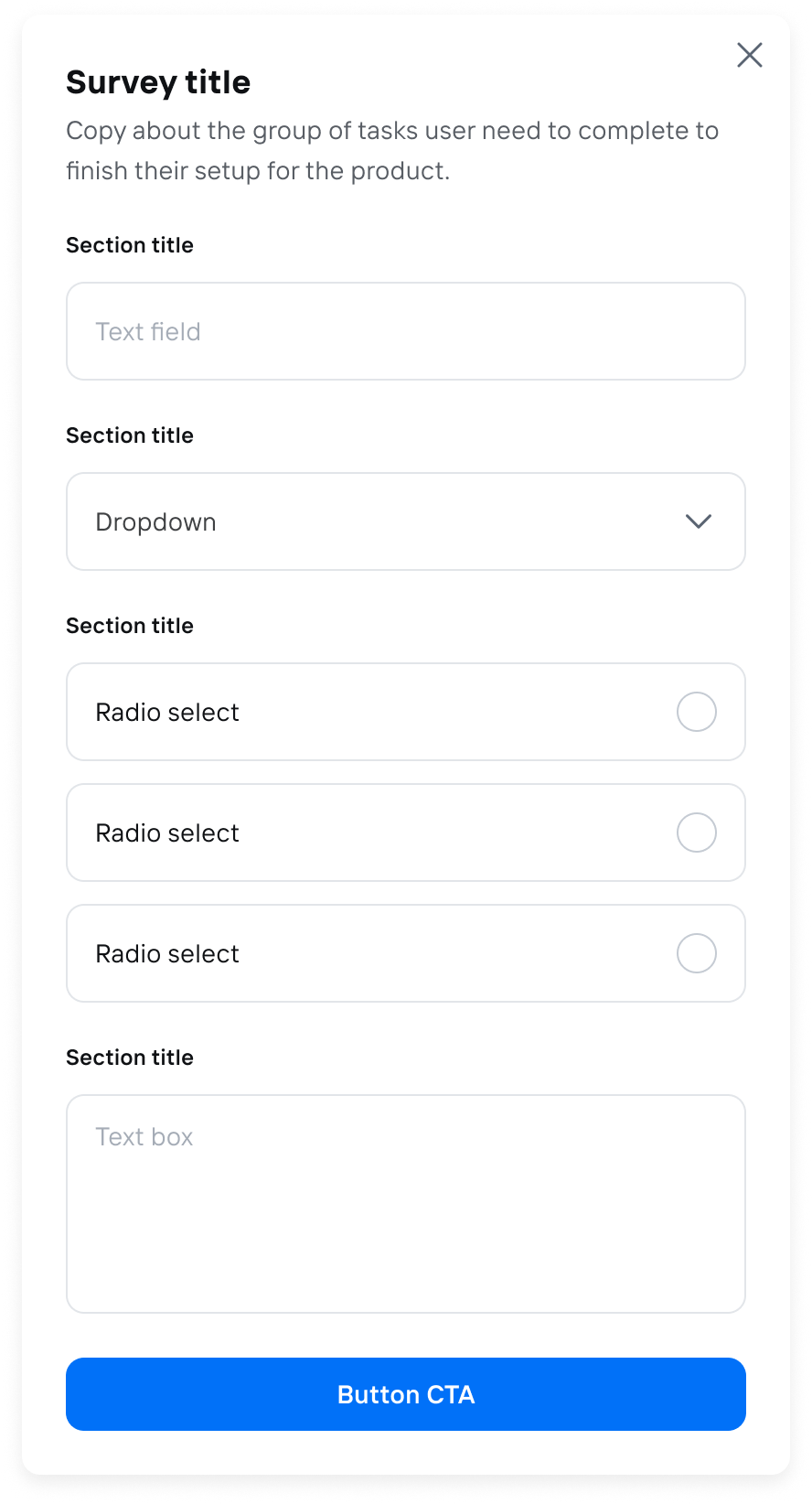
A simple <FrigadeForm/> in a popup modal
Frigade provides a set of components to help you build forms. Typical use-cases include lead collection, short user surveys, and adhoc data entry.
You can create production-ready flows for any of the components below with one click from the Components tab in the Admin Dashboard.
Related resources
Using your own components
Frigade allows you to easily use your own React components in your forms. You can either create full custom form page or use your own components for specific form elements.
Using your own input components for specific form elements
If you want to use your own input components for specific form elements, you can do so by passing in a customFormElements
prop to the <FrigadeForm/>
component.
For instance, suppose you wanted to use your own calendar picker component for a date input, you could do so in the following way:
Creating a full custom form page
If the built-in form types do not meet your use case, you can easily implement your own step in a <FrigadeForm/>
.
This is done using the customStepTypes
prop. For instance, if you wanted to build a step in a form that uses an existing React component for searching through a list of cities,
you could implement a custom form step in the following way:
Example forms
Feedback Form
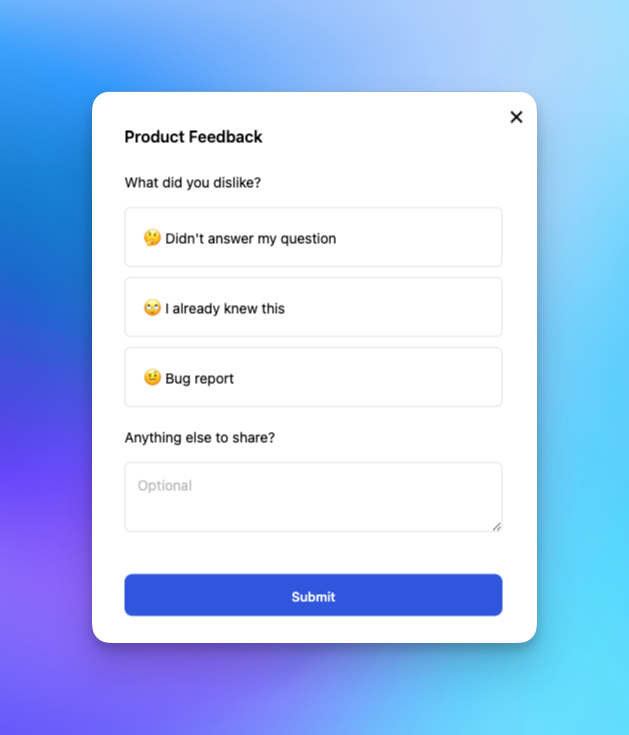
Example modal form
The code below shows the Component and Flow configuration required to render a form like the one in the screenshot above.
In this case, the form will be rendered as a modal due to the type
, but inline forms are also supported.
Additionally, this component leverages the appearance prop to customize the look and feel of the form.
Customer Support Form
data:
- id: support-page
title: Hi there, how can we help?
type: multiInput
primaryButtonTitle: Submit
props:
data:
- id: urgency
type: multipleChoice
title: Urgency
required: true
placeholder: Select an option
props:
minChoices: 1
maxChoices: 1
options:
- id: low
title: Low
- id: medium
title: Medium
- id: critical-blocker
title: Critical blocker
- id: subject
type: text
title: Subject
required: true
placeholder: Enter subject
- id: question-text
type: text
title: What is your question?
multiline: true
required: true