Advanced
Form Validation
Frigade Forms supports validation of form fields.
You can use the validation
property on any field in the multiInput
form type to validate any input. Frigade uses Zod for validation and error handling and thus the Frigade YAML schema follows the same API.
Example
The following example config.yml
contains two fields: a number field and a username field. Both have custom validation and error handling:
data:
- id: getting-started
title: Getting Started
subtitle: Let's collect some info
type: multiInput
primaryButtonTitle: Continue
props:
data:
- id: age
type: text
title: Age
required: true
validation:
type: number
requiredError: Age is required
invalidTypeError: Age must be a number
props:
- requirement: positive
message: Age must be a number
- requirement: min
value: 18
message: Sorry, you must be over 18 to use Frigade
- id: username
type: text
title: Username
required: true
validation:
type: string
requiredError: Username is required
invalidTypeError: Username must be a string
props:
- requirement: min
value: 6
message: Your username must be at least 6 characters
- requirement: max
value: 14
message: Your username must be at max 14 characters
- requirement: regex
value: "^(?=[a-zA-Z0-9._]{8,20}$)(?!.*[_.]{2})[^_.].*[^_.]$"
message: Your username is not valid
The corresponding frontend code looks like this using the <FrigadeForm />
component:
import { FrigadeForm } from '@frigade/react'
function App() {
return (
<FrigadeForm
flowId='flow_laJhda4sgJCdsCy6'
allowBackNavigation
/>
)
}
Putting it all together, this will render as follows:
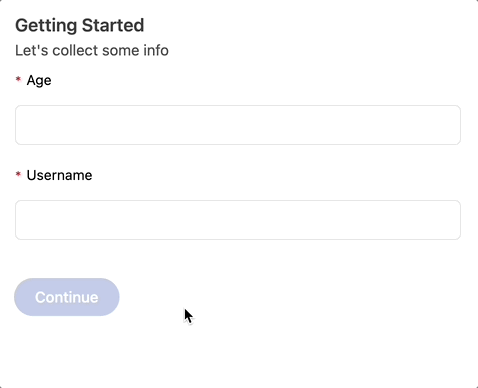
Input validation using Frigade Forms